3.2-JSP
JavaBeans
javabean是符合标准格式的Java类(就是个类,只不过把一些诸如属性、数据库连接单独拿出来作为一个类存在)。
A bean class has the same basic structure as an ordinary Java class, but should follow these rules:
-
It must be within a named package.
-
每个(不是库中的)公共方法都应该以get或set开头
-
需要被序列化并且实现了 Serializable 接口(该接口内无方法)
需要导包
Import java.io.Serializable
-
It does not usually have a main() method, but will have if some initial activity is required.
-
Must have a zero-argument (empty) constructor.
-
全为私有属性,但是要public的访问方法
-
Persistent non-boolean values should be accessed through methods called getXxx() and setXxx (). Boolean values are accessed using methods called isXxx()and setXxx().
对于布尔类型
, 可以按常规属性的规则编写getter/setter方法外, getter方法可以使用 is + ()的形式来代替
Steps to Create a JavaBean
为所需的bean功能编写程序代码(确保bean类实现了Serializable接口)
为每个属性(前端可能用到的所有属性)添加 accessor and mutator
编译bean,并尽可能将它(以及任何所需的资源文件)包装在JAR (Java Archive)文件中
public class BMIBean implements Serializable {
public static String[] bmiType = {"Normal", "Overweight", "Obese", "Extremely Obese"};
public static double[] bmiThreshold = {20.0,25.0,30.0,40.0};
private double height, weight;
public void setHeight(double h) { height = h; }
public void setWeight(double w) { weight = w; }
public double getHeight() { return height; }
public double getWeight() { return weight; }
public String calculate() throws Exception {
if (height <= 0.0)
throw new Exception("Divide by zero");
double ratio = weight/(height * height);
for (int i = 3; i >= 0; i--)
if (ratio > bmiThreshold[i])
return bmiType[i];
return "You are seriously underweight.Please seek medical advice.";
}
}
Note: No constructor given(defaultconstructor has no arguments.)
JavaBean
如何使用(在JSP中如何使用JavaBean)?
1. jsp:useBean action lets you load a bean within a JSP page
用于获取 Bean 对象。jsp:useBean 首先会在scope内查找 Bean 对象,如果 Bean 对象不存在,则创建 Bean 对象。(相当于在servlet中实例化一个类)
<jsp:useBean id=“name” class=“package.class” />
1)id
表示 Bean 实例化对象的变量名,可以在指定范围内使用该变量名。
2)class
表示类的路径 package.class。
3)scope
指定 Bean 的作用域(实例化对象的作用域),取值为:
-
page(default value)::只能在当前页面使用该 Bean 对象;(最小范围)
The bean is stored in the PageContext object for the duration of the current request
-
request:只能在一次请求范围内使用该 Bean 对象;(可以转发给别人)
The bean is stored in the HttpServletRequest object
-
session:只能在一次会话范围内使用该 Bean 对象;
The bean is stored in the HttpSession object.
-
application: The bean is stored in the ServletContext and can be accessed by other pages or servlets.
这通常意味着“实例化由class指定的类的对象,并将其绑定到由id指定的名称的变量”。
<jsp:useBean id=“bmi” class=“elem004.BMIBean” />等价于<% elem004.BMIBean bmi = new elem004.BMIBean(); %>
2.jsp:setProperty
设置 Bean 的属性值
<jsp:setProperty name = "beanName" property = "propname" value = "attributeValue"/>
name 指定需要修改属性的 Bean对象的名称;property 指定 Bean 对象的属性,即 Bean 类中的属性(成员变量);value 是要设定的属性值。也可以替换为param:将后面跟着的参数的值直接赋值给这个属性
(本质上就是调用set方法)
-
属性名区分大小写。
-
值必须用单引号或双引号括起来。
-
非容器元素应该以/>结束标记。
<jsp:setProperty name=“bmi” property=“height” value=“1.6”/> 等价于 <% bmi.setHeight(1.6); %> 前提是这个属性以及被定义才能这么用 <jsp:setProperty name=“beanName” property=“someProperty” value=‘<% request.getParameter(”someParam”) %>’ /> 简化为: <jsp:setProperty name=“beanName” property=“someProperty” param=“someParam”/> 转换到servlet: bean.someProperty=setSomeProperty(request.getParameter(”someParam”));
如果bean的属性和输入参数(前端表单里传入Http中的参数)具有完全相同的名称,则可以直接将它们关联起来。(不用写 param)
如
<jsp:setProperty name=“beanName” property=“someProperty” />
设置所有属性(有参数的属性)
<jsp:setProperty name=“beanName” property=“*” />
3.jsp:getProperty
<jsp:getProperty name = "beanName" property = "attributeName">
<jsp:getProperty name=“bmi” property=“height” />
等价于
<%= bmi.getHeight() %>
HANDLING ERRORS
<%@ page errorPage=“BMIError.jsp” %>
INCLUDING FILES IN A JSP
two ways to include other files
- Using the include directive 用includejsp指令
Used to include other JSP pages at translation time.
If file is changed, all JSP files that include it must also be updated.
引入的文件发生变化,要重新加载JSP文件
- Using the jsp:include action
- <%@ include file=“ContactSection.jsp” %>
Used to include static HTML or other text files at request time.
Included files can be modified without retranslating JSP page.
引入的文件发生变化,可以不用重新加载JSP文件
Useful for updating web information by non-programmers.
对于非程序员更新网页信息很有用
引入文件(include directive):
<%@ page import="java.util.Date" %>
<%-- The following become fields in each servlet that
results from a JSP page that includes this file. --%>
<%! private int accessCount = 0;
private Date accessDate = new Date();
private String accessHost = "<I>No previous access</I>"; %>
<P><HR>This page © 2000
<A HREF="http//www.my-company.com/">my-company.com</A>.
This page has been accessed <%= ++accessCount %>
times since server reboot. It was last accessed from
<%= accessHost %> at <%= accessDate %>.
<% accessHost = request.getRemoteHost(); %>
<% accessDate = new Date(); %>
<BODY>
<TABLE BORDER=5 ALIGN="CENTER"><TR><TH CLASS="TITLE">
Some Random Page
</TABLE>
<P> Information about our products and services.
<P> Blah, blah, blah.
<P> Yadda, yadda, yadda.
<%@ include file="ContactSection.jsp" %>
</BODY>
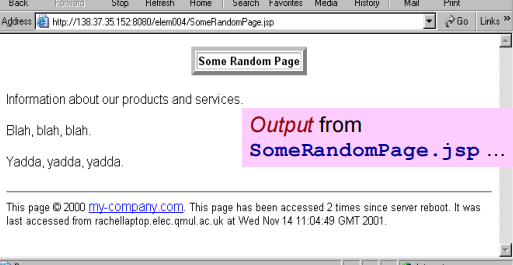
<%@ include file="ContactSection.jsp" %>
jsp:include action
<BODY>
<CENTER>
<TABLE BORDER=5>
<TR><TH CLASS="TITLE">
What's New at JspNews.com
</TABLE>
</CENTER>
<P>Here is a summary of our four most recent news stories:
<OL>
<LI> <jsp:include page="news/Item1.htm" flush="true" />
<LI> <jsp:include page="news/Item2.htm" flush="true" />
<LI> <jsp:include page="news/Item3.htm" flush="true" />
<LI> <jsp:include page="news/Item4.htm" flush="true" />
</OL>
</BODY>
<jsp:include page="news/Item1.htm" flush="true" />
Redirect and Forward
jsp:forward action
<jsp:forward page=“Relative URL” />
<% String destination = “myDestination.jsp”; %>
<jsp:forward page=“<%= destination %>” />
Forward必须在响应提交给客户端之前调用,在主体输出缓冲区被刷新之前。
如果响应缓冲区中有IllegalStateException,则在输出之前自动清除未提交的输出
所以一般来说,如果你在servlet中访问了ServletOutputStream或PrintWriter对象,你不能使用这个方法