2.4-JavaScript
JavaScript
What is JavaScript?
A scripting language most often used for client-side web development
- HTML 定义网页的内容
- CSS 规定网页的布局
- JavaScript 对网页行为进行编程
Different brands and/or different versions of browsers may support different implementations of JavaScript.
JavaScript:
• Validating forms before sending to server.
• Client-side language to manipulate all areas of the browser’s display, whereas e.g. servlets and PHPs run on server side.
• Event‐Driven Programming Model: event handlers.
• Heavyweight regular expression capability (out of scope here!)
• Interpreted language (interpreter in browser): dynamic typing.
• Functional object‐oriented language with prototypical inheritance (class free), which includes concepts of objects, arrays, prototypes …
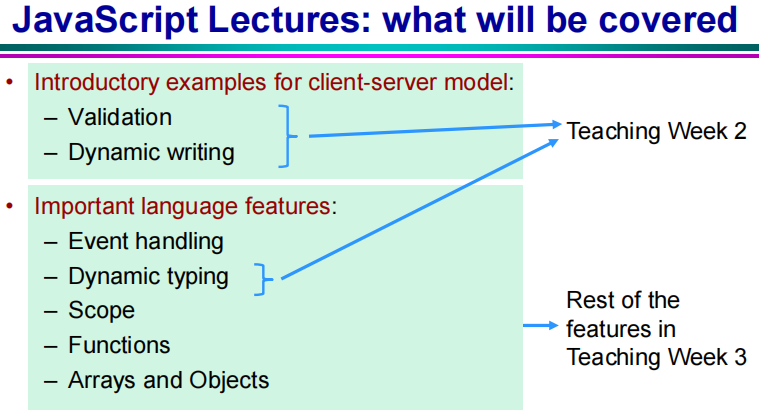
在 HTML 中,JavaScript 代码必须位于 <script>
与 </script>
标签之间。
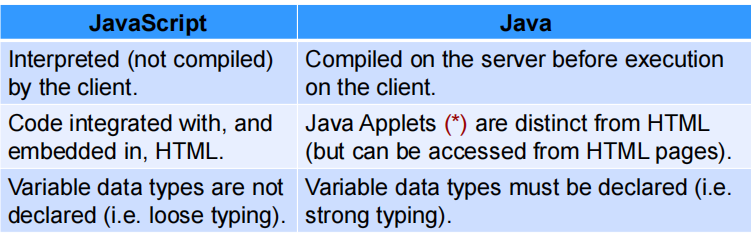
Form Validation
使用 JavaScript 来验证提交数据(客户端验证)比将数据提交到服务器再进行验证(服务器端验证)用户体验要更好,因为客户端验证发生在用户浏览器中,无需向服务器发送请求,所以速度更快,而服务器端验证,需要先将数据提交到服务器,然后服务器再将结果返回给浏览器,用户需要等待服务器响应结果才能知道哪里出了问题。
<HTML>
<HEAD>
<SCRIPT>
function validate(){
if (document.forms[0].elements[0].value==“”) {
alert(“please enter your email”);
return false;
}
return true;
}
</SCRIPT>
</HEAD>
<BODY>
<FORM method=“get” action=“someURL”
onSubmit=“return validate()”>
<input type=text name=“email”>enter your email<br>
<input type=submit value=“send”>
</FORM>
</BODY>
</HTML>
-
在 HTML 中,JavaScript 代码必须位于
<script>
与</script>
标签之间。 -
validate():在这段代码中判断输入是否为空如果为空调用alert(在浏览器中弹出一个警告框)
Variable names placed in section; can’t start with a number, have spaces or other punctuation.
<html>
<head>
<script>
var greeting=“Hello”;
</script>
</head>
<body>
<script>
document.write(“<FONT COLOR=‘magenta’>”);
document.write(greeting);
document.write(“<br>world!</FONT>”);
</script>
</body>
</html>
声明(创建) JavaScript 变量
通过 var
关键词来声明 JavaScript 变量:
var carName;
在HTML中调用JS代码的方法
-
JavaScript 代码必须位于
<script>
与</script>
标签之间。<script> document.getElementById("demo").innerHTML = "我的第一段 JavaScript"; </script>
脚本可被放置与 HTML 页面的 <body>
或 <head>
部分中,或兼而有之。
在<head>
中声明类及书写功能, 在<body>
中调用功能
-
外部脚本
脚本可放置与外部文件中:
在
<script>
标签的src
(source) 属性中设置脚本的名称:<script src="myScript.js"></script>
document.write
document.write向文档中写入HTML表达式和JavaScript的代码。
document.writeln()输出结果相当于document.write()输出之后加空格(html源码中换行)
– writeln break on the page need to add adds a return character after the text string, but to get a line <br>
并不会换行
使用header也可以自动换行
– document.write(“
Top level header
”);– document.write(“
Lowest level header
”);文本中可以写入HTML标签
If just want a line break:
– document.write('<br>'
);
Dynamic Typing: type is defined by the literal value you assign to it
javaScript 拥有动态类型。这意味着相同的变量可用作不同的类型(取决于你赋的值):
值类型(基本类型):字符串(String)、数字(Number)、布尔(Boolean)、空(Null)、未定义(Undefined)、Symbol。
引用数据类型(对象类型):对象(Object)、数组(Array)、函数(Function)
变量的数据类型可以使用 typeof 操作符来查看:
typeof "John" // 返回 string
typeof 3.14 // 返回 number
typeof false // 返回 boolean
typeof [1,2,3,4] // 返回 object
x=null; to identify a deliberately null or empty reference
If x is undefined, it has not been set a value (MUST define a value for a const).
Implicit conversion between types
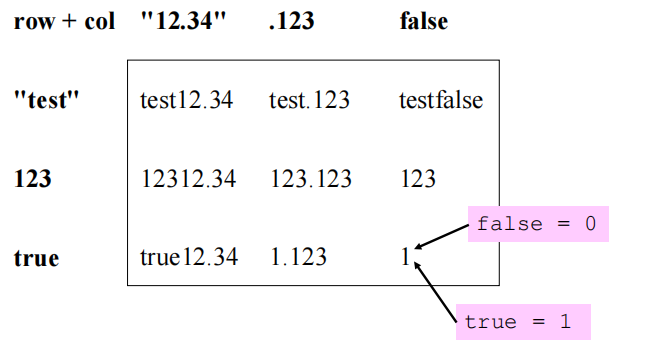
<head>
<script type=“text/javascript”>
x = 0xF;
y = true;
z = false;
</script>
</head>
<body>
<script type=“text/javascript”>
document.write("x+y = ");
document.write(x+y);
document.write("x+z = ");
document.write(x+z);
</script>
</body>
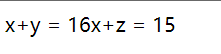
a = 1;
b = “20”;
c = a + b; // 120 b+a 201
c = a + (b-0); // 21
c = a + “”; //The empty string, forcing conversion into a string.
The number 0. JavaScript prefers numeric conversion with the minus (and prefers string conversion with +).
a+b=120
b+a=201
x = 7;
y = 4;
sum = x + y;
document.writeln(“<H1>x + y = ” + sum + “ </H1>”);
document.writeln(“<H1>x + y = ” + x+y + “ </H1>”);
document.writeln(“<H1>x + y = ” + (x+y) + “ </H1>”);
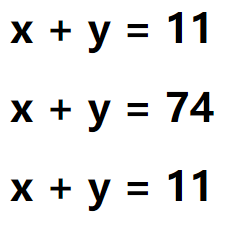
- 2.0.0.1.1. Implicit conversion between types
- 2.0.0.1.2. 标签的属性</h5> <p>1、src</p> <blockquote> <p>定义引用外部脚本的URI,这可以用来代替直接在文档中嵌入脚本。指定了 src 属性的script元素标签内不应该再有嵌入的脚本。</p> </blockquote> <pre class="line-numbers language-xml" data-language="xml"><code class="language-xml"><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>script</span> <span class="token attr-name">src</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token punctuation">"</span>file.js<span class="token punctuation">"</span></span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>script</span><span class="token punctuation">></span></span><span aria-hidden="true" class="line-numbers-rows"><span></span></span></code></pre> <p>2、type</p> <blockquote> <p>该属性定义script元素包含或src引用的脚本语言。属性的值为MIME类型;</p> </blockquote> <p>只能是下面几种方式:</p> <ul> <li><strong>text/javascript(默认)</strong></li> <li>text/ecmascript</li> <li>application/javascript</li> <li>application/ecmascript</li> </ul> <h5 id="Quotes-within-strings"><strong>Quotes within strings</strong></h5> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript">document<span class="token punctuation">.</span><span class="token function">write</span><span class="token punctuation">(</span>“He said<span class="token punctuation">,</span> \”That’s mine\” ”<span class="token punctuation">)</span><span class="token punctuation">;</span> document<span class="token punctuation">.</span><span class="token function">write</span><span class="token punctuation">(</span>‘She said<span class="token punctuation">,</span> ”No it\’s not” ’<span class="token punctuation">)</span><span class="token punctuation">;</span> document<span class="token punctuation">.</span><span class="token function">write</span><span class="token punctuation">(</span>‘He said<span class="token punctuation">,</span> ”That\’s mine” ’<span class="token punctuation">)</span><span class="token punctuation">;</span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span></span></code></pre> <p><img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture%2Fimage-20221101225055253.png" alt="image-20221101225055253"></p> <p>这啥意思啊</p> <h5 id="Comparison-Operators"><strong>Comparison Operators</strong></h5> <ul> <li> <p><strong>==</strong> and <strong>!=</strong></p> <p>do perform type conversion before comparison</p> <p><strong>“5”==5</strong> is <em>true</em></p> </li> <li> <p><strong>===</strong> and <strong>!==</strong></p> <p>do not perform type conversion</p> <p><strong>“5”===5</strong> is <em>false</em></p> </li> </ul> <p><img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture%2Fimage-20221102104308480.png" alt="image-20221102104308480"></p> <h5 id="Explicit-Conversion-Functions"><strong>Explicit Conversion Functions</strong></h5> <ul> <li> <p><strong>eval()</strong> takes a string parameter and evaluates it.</p> <p><strong>x = eval(“123.35*67”);</strong></p> <p>**eval(“x=1; y=2; x+y”);**Error if string doesn’t evaluate</p> </li> </ul> <p>to a numeric value = <strong>NaN</strong></p> <p>如果参数是字符串,并且字符串中是表达式,则eval()方法会计算字符串中的表达式,返回该表达式计算的结果。</p> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript"><span class="token function">eval</span><span class="token punctuation">(</span><span class="token string">"'true'"</span><span class="token punctuation">)</span><span class="token punctuation">;</span><span class="token comment">//返回字符串"true"</span> <span class="token function">eval</span><span class="token punctuation">(</span><span class="token string">"ture"</span><span class="token punctuation">)</span><span class="token punctuation">;</span><span class="token comment">//返回Boolean类型的true</span> <span class="token function">eval</span><span class="token punctuation">(</span><span class="token string">"1"</span><span class="token punctuation">)</span><span class="token punctuation">;</span><span class="token comment">//返回整数1</span> <span class="token function">eval</span><span class="token punctuation">(</span><span class="token string">"[0,1,2]"</span><span class="token punctuation">)</span><span class="token punctuation">;</span><span class="token comment">//返回数组[0,1,2]</span> <span class="token function">eval</span><span class="token punctuation">(</span><span class="token string">"2+2"</span><span class="token punctuation">)</span><span class="token punctuation">;</span><span class="token comment">//返回整数4</span> <span class="token function">eval</span><span class="token punctuation">(</span><span class="token string">"2+'2'"</span><span class="token punctuation">)</span><span class="token punctuation">;</span><span class="token comment">//返回字符串"22"</span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span><span></span><span></span><span></span></span></code></pre> <ul> <li> <p>parseInt() returns the first integer in a string; if no integer returns NaN <em>将一个string转换成整数,只取第一个整数</em></p> <p>parseInt(“123xyz”) returns 123 as an integer</p> <p>parseInt(“xyz”) returns NaN(<strong>NaN 属性是代表非数字值的特殊值。</strong>)</p> <p>语法:parseInt(<em>string</em>,radix);</p> <ul> <li>string:要被解析的值。如果参数不是一个字符串,则将其转换为字符串(toString)。字符串开头的空白符将会被忽略。</li> <li>*radix:可选。*从 <code>2</code> 到 <code>36</code>,表示被解析的值的进制。例如说指定 <code>10</code> 就等于指定十进位。</li> </ul> </li> <li> <p>parseFloat()</p> <p>parseFloat(“123.45xyz”) returns 123.45</p> </li> <li> <p>isNaN() returns true if input is not a number(值)</p> </li> </ul> <p>isNaN(“4”) returns <strong>false</strong></p> <p>isNaN(4) returns false</p> <p>isNaN(parseInt(“4”)) returns false</p> <p>isNaN(parseInt(“four”)) returns true</p> <p><code>isNaN</code>只对数值有效,如果传入其他值,会被先转成数值。 <strong><code>isNaN</code>为<code>true</code>的值,有可能不是<code>NaN</code>,而是一个字符串</strong></p> <h5 id="implicit-conversion">implicit conversion</h5> <p>JavaScript 中,表达式中包含以下运算符时,会发生隐式类型转换:</p> <p>算术运算符:加(+)、减(-)、乘(*)、除(/)、取模(%);逻辑运算符:逻辑与(&&)、逻辑或(||)、逻辑非(!);字符串运算符:+、+=。</p> <p>隐式转换规律:</p> <ul> <li>'string + number’字符串加数字,数字会转换为字符串;</li> <li>‘number - string’ 数字减字符串,字符串会转换为数字,<strong>如果字符串无法转换为数字(如"abc"、“JavaScript”),则会转换为 NaN;</strong></li> <li>'string - number’字符串减数字,字符串会转换为数字,如果字符串无法转换为数字,则会转换为 NaN;即’➖’优先向数字转换。<br> 乘、除运算时,也会先将字符串转换为数字。<br> <strong>当字符串和数字同时存在时,除了加号运算符转化为字符串,其他减、乘和除都优先转化为数字。</strong></li> </ul> <p>加号:如果有字符串,把所有都转成字符串然后拼接;如果没有直接转数值</p> <p>减号:直接转数值</p> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript"><span class="token operator"><</span><span class="token constant">HTML</span><span class="token operator">></span> <span class="token operator"><</span><span class="token constant">HEAD</span><span class="token operator">></span><span class="token operator"><</span><span class="token constant">SCRIPT</span> type<span class="token operator">=</span><span class="token string">"text/javascript"</span><span class="token operator">></span> <span class="token keyword">function</span> <span class="token function">updateOrder</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span> <span class="token keyword">const</span> cakePrice <span class="token operator">=</span> <span class="token number">1</span><span class="token punctuation">;</span> <span class="token keyword">const</span> bunPrice <span class="token operator">=</span> <span class="token number">0.5</span><span class="token punctuation">;</span> <span class="token keyword">var</span> noCakes <span class="token operator">=</span> document<span class="token punctuation">.</span><span class="token function">getElementById</span><span class="token punctuation">(</span><span class="token string">"cake"</span><span class="token punctuation">)</span><span class="token punctuation">.</span>value<span class="token punctuation">;</span> <span class="token keyword">var</span> noBuns <span class="token operator">=</span> document<span class="token punctuation">.</span><span class="token function">getElementById</span><span class="token punctuation">(</span><span class="token string">"bun"</span><span class="token punctuation">)</span><span class="token punctuation">.</span>value<span class="token punctuation">;</span> document<span class="token punctuation">.</span><span class="token function">getElementById</span><span class="token punctuation">(</span><span class="token string">"count"</span><span class="token punctuation">)</span><span class="token punctuation">.</span>value <span class="token operator">=</span> <span class="token function">parseInt</span><span class="token punctuation">(</span>noCakes<span class="token punctuation">,</span> <span class="token number">10</span><span class="token punctuation">)</span> <span class="token operator">+</span> <span class="token function">parseInt</span><span class="token punctuation">(</span>noBuns<span class="token punctuation">,</span> <span class="token number">10</span><span class="token punctuation">)</span><span class="token punctuation">;</span> document<span class="token punctuation">.</span><span class="token function">getElementById</span><span class="token punctuation">(</span><span class="token string">"total"</span><span class="token punctuation">)</span><span class="token punctuation">.</span>value <span class="token operator">=</span> <span class="token string">"£"</span> <span class="token operator">+</span> <span class="token punctuation">(</span>cakePrice<span class="token operator">*</span>noCakes <span class="token operator">+</span> bunPrice<span class="token operator">*</span>noBuns<span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token punctuation">}</span> <span class="token keyword">function</span> <span class="token function">placeOrder</span><span class="token punctuation">(</span><span class="token parameter">form</span><span class="token punctuation">)</span> <span class="token punctuation">{</span> form<span class="token punctuation">.</span><span class="token function">submit</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token punctuation">}</span> <span class="token operator"><</span><span class="token operator">/</span><span class="token constant">SCRIPT</span><span class="token operator">></span><span class="token operator"><</span><span class="token operator">/</span><span class="token constant">HEAD</span><span class="token operator">></span> <span class="token operator"><</span><span class="token constant">BODY</span><span class="token operator">></span><span class="token operator"><</span><span class="token constant">FORM</span> method<span class="token operator">=</span><span class="token string">"get"</span> action<span class="token operator">=</span><span class="token string">"someURL"</span><span class="token operator">></span> <span class="token operator"><</span><span class="token constant">H3</span><span class="token operator">></span>Cakes Cost £<span class="token number">1</span> each<span class="token punctuation">;</span> Buns 50p each<span class="token operator"><</span><span class="token operator">/</span><span class="token constant">H3</span><span class="token operator">></span> <span class="token operator"><</span>input type<span class="token operator">=</span>text name<span class="token operator">=</span><span class="token string">"cakeNo"</span> id<span class="token operator">=</span><span class="token string">"cake"</span> onchange<span class="token operator">=</span><span class="token string">"updateOrder()"</span><span class="token operator">></span>enter no<span class="token punctuation">.</span> cakes<span class="token operator"><</span>br<span class="token operator">></span> <span class="token operator"><</span>input type<span class="token operator">=</span>text name<span class="token operator">=</span><span class="token string">"bunNo"</span> id<span class="token operator">=</span><span class="token string">"bun"</span> onchange<span class="token operator">=</span><span class="token string">"updateOrder()"</span><span class="token operator">></span>enter no<span class="token punctuation">.</span> buns<span class="token operator"><</span>br<span class="token operator">></span> <span class="token operator"><</span>input type<span class="token operator">=</span>text name<span class="token operator">=</span><span class="token string">"count"</span> id<span class="token operator">=</span><span class="token string">"count"</span><span class="token operator">></span>number <span class="token keyword">of</span> units<span class="token operator"><</span>br<span class="token operator">></span> <span class="token operator"><</span>input type<span class="token operator">=</span>text name<span class="token operator">=</span><span class="token string">"total"</span> id<span class="token operator">=</span><span class="token string">"total"</span><span class="token operator">></span>Total Price<span class="token operator"><</span>br<span class="token operator">></span> <span class="token operator"><</span>input type<span class="token operator">=</span>button value<span class="token operator">=</span><span class="token string">"send"</span> onClick<span class="token operator">=</span><span class="token string">"placeOrder(this.form)"</span><span class="token operator">></span> <span class="token operator"><</span><span class="token operator">/</span><span class="token constant">FORM</span><span class="token operator">></span><span class="token operator"><</span><span class="token operator">/</span><span class="token constant">BODY</span><span class="token operator">></span> <span class="token operator"><</span><span class="token operator">/</span><span class="token constant">HTML</span><span class="token operator">></span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span></span></code></pre> <p><img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture%2Fimage-20221102112548088.png" alt="image-20221102112548088"></p> <h3 id="Document-Object-Model"><strong>Document Object Model</strong></h3> <p>Provides structural representation of a document, so its content and visual presentation can be modified</p> <p><img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture/image-20221108214606514.png" alt="image-20221108214606514"></p> <p>Navigating to <em>elements</em> and accessing user input:</p> <p>获取元素的方法:</p> <p><img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture/image-20221108215034741.png" alt="image-20221108215034741"></p> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript">d <span class="token operator">=</span> document<span class="token punctuation">.</span><span class="token function">getElementById</span><span class="token punctuation">(</span>“usrname"<span class="token punctuation">)</span><span class="token punctuation">;</span><span aria-hidden="true" class="line-numbers-rows"><span></span></span></code></pre> <p>如果有多个值返回,返回类型为数组</p> <h3 id="Window">Window</h3> <p><img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture/image-20221108221200869.png" alt="image-20221108221200869"></p> <ul> <li><strong>alert()</strong> 方法: 弹出信息(window 类中方法)</li> </ul> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript">window<span class="token punctuation">.</span><span class="token function">alert</span><span class="token punctuation">(</span>“Please enter name<span class="token operator">:</span>”<span class="token punctuation">)</span><span class="token punctuation">;</span><span aria-hidden="true" class="line-numbers-rows"><span></span></span></code></pre> <p>windows.confirm()弹出带有确认和取消的对话框,返回true/false</p> <ul> <li> <p>confirm(“Are you sure you want to submit?”)</p> </li> <li> <p>setInterval() 以某个周期调用函数</p> <pre class="line-numbers language-js" data-language="js"><code class="language-js"><span class="token function">setInterval</span><span class="token punctuation">(</span>code<span class="token punctuation">,</span> milliseconds<span class="token punctuation">)</span><span class="token punctuation">;</span><span aria-hidden="true" class="line-numbers-rows"><span></span></span></code></pre> </li> <li> <p>**setTimeout()**delay before execution</p> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript"><span class="token function">setTimeout</span><span class="token punctuation">(</span>要执行的代码<span class="token punctuation">,</span> 等待的毫秒数<span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token function">setTimeout</span><span class="token punctuation">(</span><span class="token function">myFunc</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">,</span> <span class="token number">1</span><span class="token punctuation">)</span> – delay <span class="token number">1</span> ms <span class="token function">setTimeout</span><span class="token punctuation">(</span><span class="token function">myFunc</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">,</span><span class="token number">1000</span><span class="token punctuation">)</span> – delay <span class="token number">1</span> second<span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span></span></code></pre> </li> </ul> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript"><span class="token operator"><</span><span class="token constant">HTML</span><span class="token operator">></span><span class="token operator"><</span><span class="token constant">HEAD</span><span class="token operator">></span><span class="token operator"><</span><span class="token constant">TITLE</span><span class="token operator">></span><span class="token constant">DHTML</span> Event Model <span class="token operator">-</span> <span class="token constant">ONLOAD</span><span class="token operator"><</span><span class="token operator">/</span><span class="token constant">TITLE</span><span class="token operator">></span> <span class="token operator"><</span><span class="token constant">SCRIPT</span><span class="token operator">></span> <span class="token keyword">var</span> seconds <span class="token operator">=</span> <span class="token number">0</span><span class="token punctuation">;</span> <span class="token keyword">function</span> <span class="token function">startTimer</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span> window<span class="token punctuation">.</span><span class="token function">setInterval</span><span class="token punctuation">(</span><span class="token string">"updateTime()"</span><span class="token punctuation">,</span> <span class="token number">1000</span> <span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token punctuation">}</span> <span class="token keyword">function</span> <span class="token function">updateTime</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span> seconds<span class="token operator">++</span><span class="token punctuation">;</span> soFar<span class="token punctuation">.</span>innerText <span class="token operator">=</span> seconds<span class="token punctuation">;</span> <span class="token punctuation">}</span> <span class="token operator"><</span><span class="token operator">/</span><span class="token constant">SCRIPT</span><span class="token operator">></span><span class="token operator"><</span><span class="token operator">/</span><span class="token constant">HEAD</span><span class="token operator">></span> <span class="token operator"><</span><span class="token constant">BODY</span> <span class="token constant">ONLOAD</span> <span class="token operator">=</span> <span class="token string">"startTimer()"</span><span class="token operator">></span> <span class="token operator"><</span><span class="token constant">P</span><span class="token operator">></span>Seconds you have spent viewing <span class="token keyword">this</span> page so far<span class="token operator">:</span> <span class="token operator"><</span><span class="token constant">A</span> <span class="token constant">ID</span> <span class="token operator">=</span> <span class="token string">"soFar"</span> <span class="token constant">STYLE</span> <span class="token operator">=</span> <span class="token string">"font-weight: bold"</span><span class="token operator">></span><span class="token number">0</span><span class="token operator"><</span><span class="token operator">/</span><span class="token constant">A</span><span class="token operator">></span><span class="token operator"><</span><span class="token operator">/</span><span class="token constant">P</span><span class="token operator">></span> <span class="token operator"><</span><span class="token operator">/</span><span class="token constant">BODY</span><span class="token operator">></span><span class="token operator"><</span><span class="token operator">/</span><span class="token constant">HTML</span><span class="token operator">></span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span></span></code></pre> <p>之所以第7行直接使用id名可以使用:如果一个元素拥有ID属性,那么ID属性的属性值就会成为window对象的属性名.</p> <ul> <li> <p><strong>clearInterval()</strong> 停止执行</p> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript">myVar <span class="token operator">=</span> <span class="token function">setInterval</span><span class="token punctuation">(</span><span class="token function">myFunc</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">,</span> <span class="token number">1000</span><span class="token punctuation">)</span> <span class="token function">clearInterval</span><span class="token punctuation">(</span>myVar<span class="token punctuation">)</span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span></span></code></pre> </li> <li> <p><strong>clearTimeout()</strong> 停止执行</p> </li> </ul> <h3 id="Event-Driven-Programming"><strong>Event-Driven Programming</strong></h3> <p>Web browser generates an event whenever anything ‘interesting’ occurs.</p> <p>JavaScript application registers an <strong>event handler</strong>.</p> <p>方法一:input标签里对应事件元素<img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture/image-20221108213542145.png" alt=""></p> <p><img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture/ad7402a19b5f4d87be88d0cdafd47ad.jpg" alt="ad7402a19b5f4d87be88d0cdafd47ad"></p> <img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture/image-20221108215132875.png" alt="image-20221108215132875" style="zoom:80%;" /> <p>如:</p> <pre class="line-numbers language-html" data-language="html"><code class="language-html"><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>input</span> <span class="token attr-name">type</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“button”</span> <span class="token attr-name">value</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“push”</span> <span class="token special-attr"><span class="token attr-name">onclick</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token value javascript language-javascript"><span class="token function">validate</span><span class="token punctuation">(</span><span class="token punctuation">)</span></span></span></span><span class="token punctuation">></span></span><span aria-hidden="true" class="line-numbers-rows"><span></span></span></code></pre> <p>其中Calls <strong>validate()</strong> function defined in <strong><head></strong></p> <p><strong>document.forms[0].submit()</strong></p> <pre class="line-numbers language-html" data-language="html"><code class="language-html"><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>form</span> <span class="token attr-name">action</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“someURL”</span> <span class="token special-attr"><span class="token attr-name">onSubmit</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token value javascript language-javascript">“<span class="token keyword">return</span></span></span></span> <span class="token attr-name">validate()”</span><span class="token punctuation">></span></span> // textboxes etc <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>input</span> <span class="token attr-name">type</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“submit”</span> <span class="token attr-name">value</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“send”</span><span class="token punctuation">></span></span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span></span></code></pre> <p>onSubmit=“return validate()”:当**validate()**返回true,submit。否则不提交</p> <pre class="line-numbers language-js" data-language="js"><code class="language-js">onsubmit<span class="token operator">=</span><span class="token string">"return validate()"</span> 相当于: Form<span class="token punctuation">.</span>prototype<span class="token punctuation">.</span><span class="token function-variable function">onsubmit</span> <span class="token operator">=</span> <span class="token keyword">function</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span> <span class="token keyword">return</span> <span class="token function">validate</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token punctuation">}</span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span><span></span><span></span></span></code></pre> <p><strong>onSubmit=validate()</strong> 总是提交(submit的机制)</p> <p>可以使用button加onclick解决(其中onclick对应的函数中要用提交语句document.Formname.submit();)</p> <p>只要进行submit,就必须在form标签里添加action和method,此外如果有表单验证函数,必须返回Boolean值</p> <pre class="line-numbers language-html" data-language="html"><code class="language-html"><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>html</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>head</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>script</span> <span class="token attr-name">type</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“text/javascript”</span><span class="token punctuation">></span></span><span class="token script"><span class="token language-javascript"> <span class="token keyword">function</span> <span class="token function">validate</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span> <span class="token keyword">if</span> <span class="token punctuation">(</span>document<span class="token punctuation">.</span>forms<span class="token punctuation">[</span><span class="token number">0</span><span class="token punctuation">]</span><span class="token punctuation">.</span>usrname<span class="token punctuation">.</span>value<span class="token operator">==</span>“”<span class="token punctuation">)</span> <span class="token punctuation">{</span> <span class="token function">alert</span><span class="token punctuation">(</span>“please enter name”<span class="token punctuation">)</span><span class="token punctuation">;</span> document<span class="token punctuation">.</span>forms<span class="token punctuation">[</span><span class="token number">0</span><span class="token punctuation">]</span><span class="token punctuation">.</span>usrname<span class="token punctuation">.</span><span class="token function">focus</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span><span class="token comment">//提交时,光标定位在第一个输入框</span> document<span class="token punctuation">.</span>forms<span class="token punctuation">[</span><span class="token number">0</span><span class="token punctuation">]</span><span class="token punctuation">.</span>usrname<span class="token punctuation">.</span>value<span class="token operator">=</span>“please enter name”<span class="token punctuation">;</span> document<span class="token punctuation">.</span><span class="token function">getElementById</span><span class="token punctuation">(</span>“a”<span class="token punctuation">)</span><span class="token punctuation">.</span>innerHTML<span class="token operator">=</span>“please enter name above”<span class="token punctuation">;</span> <span class="token comment">//inner HTML插入文本到目标标签间</span> <span class="token punctuation">}</span> <span class="token punctuation">}</span> </span></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>script</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>head</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>body</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>font</span> <span class="token attr-name">face</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“arial”</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>h1</span><span class="token punctuation">></span></span>Please enter details<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>h1</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>form</span><span class="token punctuation">></span></span> Name <span class="token entity named-entity" title=" "> </span> //设置空格 <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>input</span> <span class="token attr-name">type</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“text”</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“usrname”</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>br</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>font</span> <span class="token attr-name">color</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“red”</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>p</span> <span class="token attr-name">id</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token punctuation">"</span>a<span class="token punctuation">"</span></span><span class="token punctuation">></span></span> 插入位置 <span class="token tag"><span class="token tag"><span class="token punctuation"></</span>p</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>font</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>font</span> <span class="token attr-name">face</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“arial”</span><span class="token punctuation">></span></span> Email <span class="token entity named-entity" title=" "> </span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>input</span> <span class="token attr-name">type</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“text”</span> <span class="token attr-name">name</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“email”</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>br</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>br</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>input</span> <span class="token attr-name">type</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“button”</span> <span class="token attr-name">value</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>“Send”</span> <span class="token special-attr"><span class="token attr-name">onclick</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token value javascript language-javascript"><span class="token function">“validate</span><span class="token punctuation">(</span><span class="token punctuation">)</span>”</span></span></span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>form</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"></</span>body</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>html</span><span class="token punctuation">></span></span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span></span></code></pre> <p>可以用document.form[0]/document.formname/getElementBy…()方法 定位标签</p> <img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture/image-20221108220545701.png" alt="image-20221108220545701" style="zoom:67%;" /> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript"><span class="token operator"><</span><span class="token constant">HTML</span><span class="token operator">></span><span class="token operator"><</span><span class="token constant">HEAD</span><span class="token operator">></span><span class="token operator"><</span><span class="token constant">TITLE</span><span class="token operator">></span>Object Model<span class="token operator"><</span><span class="token operator">/</span><span class="token constant">TITLE</span><span class="token operator">></span> <span class="token operator"><</span><span class="token constant">SCRIPT</span><span class="token operator">></span> <span class="token keyword">function</span> <span class="token function">start</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span> <span class="token function">alert</span><span class="token punctuation">(</span>pText<span class="token punctuation">.</span>innerText<span class="token punctuation">)</span><span class="token punctuation">;</span> pText<span class="token punctuation">.</span>innerText <span class="token operator">=</span> “Thanks <span class="token keyword">for</span> coming<span class="token punctuation">.</span>”<span class="token punctuation">;</span> <span class="token punctuation">}</span> <span class="token operator"><</span><span class="token operator">/</span><span class="token constant">SCRIPT</span><span class="token operator">></span><span class="token operator"><</span><span class="token operator">/</span><span class="token constant">HEAD</span><span class="token operator">></span> <span class="token operator"><</span><span class="token constant">BODY</span> <span class="token constant">ONLOAD</span> <span class="token operator">=</span> <span class="token function">“start</span><span class="token punctuation">(</span><span class="token punctuation">)</span>”<span class="token operator">></span> <span class="token operator"><</span><span class="token constant">P</span> <span class="token constant">ID</span> <span class="token operator">=</span> “pText”<span class="token operator">></span>Welcome to our Web page<span class="token operator">!</span><span class="token operator"><</span><span class="token operator">/</span><span class="token constant">P</span><span class="token operator">></span> <span class="token operator"><</span><span class="token operator">/</span><span class="token constant">BODY</span><span class="token operator">></span><span class="token operator"><</span><span class="token operator">/</span><span class="token constant">HTML</span><span class="token operator">></span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span></span></code></pre> <p><strong>pText.innerText</strong> or<strong>document.getElementById(“pText”).innerHTML</strong> 可以查找文本或替换文本</p> <h3 id="STRINGS"><strong>STRINGS</strong></h3> <p><img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture/image-20221108220958551.png" alt="image-20221108220958551"></p> <p>常用:</p> <p><strong>if (ph.charAt(i)>=‘A’ && ph.charAt(i) <= ‘Z’)</strong></p> <p><strong>if (ph.length < 9)</strong></p> <p><strong>if (ph.substring(0, 1) == ‘,’)</strong></p> <p><strong>if (ph.indexOf(‘@’) == 0)</strong></p> <h3 id="Functions-2"><strong>Functions</strong></h3> <p>function 语句用于声明一个函数。</p> <p>在 JavaScript 中,函数是对象,函数也有属性和方法</p> <p>返回直接用return,参数直接写名字不加类型</p> <p>Local call: call a function directly: <strong>var x = square(4);</strong></p> <p>Callback: function is called in response to something happening: <strong>events;</strong></p> <h3 id="Arrays"><strong>Arrays</strong></h3> <p><img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture/image-20221108222545924.png" alt="image-20221108222545924"></p> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript">list <span class="token operator">=</span> <span class="token punctuation">[</span>“Karen”<span class="token punctuation">,</span> “John”<span class="token punctuation">,</span> “Stefan”<span class="token punctuation">]</span><span class="token punctuation">;</span> <span class="token keyword">var</span> list <span class="token operator">=</span> <span class="token punctuation">[</span>“Karen”<span class="token punctuation">,</span> <span class="token number">4</span><span class="token punctuation">,</span> “Stefan”<span class="token punctuation">]</span><span class="token punctuation">;</span> <span class="token comment">// mixed array</span> <span class="token keyword">var</span> emptyList <span class="token operator">=</span> <span class="token punctuation">[</span><span class="token punctuation">]</span><span class="token punctuation">;</span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span></span></code></pre> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript"><span class="token keyword">var</span> myArray <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Array</span><span class="token punctuation">(</span>length<span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token comment">// array constructor</span> employee <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Array</span><span class="token punctuation">(</span><span class="token number">3</span><span class="token punctuation">)</span><span class="token punctuation">;</span> employee<span class="token punctuation">[</span><span class="token number">0</span><span class="token punctuation">]</span> <span class="token operator">=</span> “Karen”<span class="token punctuation">;</span> employee<span class="token punctuation">[</span><span class="token number">1</span><span class="token punctuation">]</span> <span class="token operator">=</span> “John”<span class="token punctuation">;</span> employee<span class="token punctuation">[</span><span class="token number">2</span><span class="token punctuation">]</span> <span class="token operator">=</span> “Stefan”<span class="token punctuation">;</span> document<span class="token punctuation">.</span><span class="token function">write</span><span class="token punctuation">(</span>employee<span class="token punctuation">[</span><span class="token number">0</span><span class="token punctuation">]</span><span class="token punctuation">)</span><span class="token punctuation">;</span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span><span></span><span></span><span></span></span></code></pre> <h4 id="变长:Sparse-Arrays">变长:<strong>Sparse Arrays</strong></h4> <p><strong>new Array()</strong></p> <p>Length is <strong>0</strong> initially; automatically extends when new elements are initialised</p> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript">employee <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Array</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span> employee<span class="token punctuation">[</span><span class="token number">5</span><span class="token punctuation">]</span> <span class="token operator">=</span> “John”<span class="token punctuation">;</span> <span class="token comment">//length now becomes 6</span> employee<span class="token punctuation">[</span><span class="token number">100</span><span class="token punctuation">]</span> <span class="token operator">=</span> “Rachel”<span class="token punctuation">;</span> <span class="token comment">//length now becomes 101</span> <span class="token comment">//length is found as employee.length</span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span><span></span><span></span><span></span></span></code></pre> <p><strong>No array bounds errors.</strong></p> <h4 id="字符串索引">字符串索引</h4> <p>当我们为js数组添加字符串下标的时候,其实是为该<code>数组对象</code>添加了一个属性, 而添加到数组对象上的属性,并不影响该数组对象的<strong>length属性</strong>,所以在上图中打印数组,看到length为0。</p> <p><strong>如果索引为字符串不会改变length</strong></p> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript"><span class="token constant">A</span> <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Object</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token constant">A</span><span class="token punctuation">[</span>“red”<span class="token punctuation">]</span> <span class="token operator">=</span> <span class="token number">127</span><span class="token punctuation">;</span> <span class="token constant">A</span><span class="token punctuation">[</span>“green”<span class="token punctuation">]</span> <span class="token operator">=</span> <span class="token number">255</span><span class="token punctuation">;</span> <span class="token constant">A</span><span class="token punctuation">[</span>“blue”<span class="token punctuation">]</span> <span class="token operator">=</span> <span class="token number">64</span><span class="token punctuation">;</span> <span class="token comment">//等同于:</span> <span class="token constant">A</span> <span class="token operator">=</span> <span class="token keyword">new</span> <span class="token class-name">Object</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token constant">A</span><span class="token punctuation">.</span>red <span class="token operator">=</span> <span class="token number">127</span><span class="token punctuation">;</span> <span class="token constant">A</span><span class="token punctuation">.</span>green <span class="token operator">=</span> <span class="token number">255</span><span class="token punctuation">;</span> <span class="token constant">A</span><span class="token punctuation">.</span>blue <span class="token operator">=</span> <span class="token number">64</span><span class="token punctuation">;</span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span></span></code></pre> <p><strong>typeof(new array())</strong> 返回object</p> <h3 id="Scope"><strong>Scope</strong></h3> <p>JavaScript has function scope, so variables defined within a function are visible everywhere in the function</p> <p>declare at the start/top of the function body.</p> <p>Inner functions can access actual parameters and variables (not copies) of their outer functions</p> <ul> <li> <p>funcation 中的 declared variable have localscope</p> </li> <li> <p>Non-declared variable have gloable scope</p> </li> </ul> <p>如果在函数中我们没有使用var语句声明变量,默认为gloable scope,用了就是local scope</p> <p>函数外部的都为gloable scope</p> <h3 id="Cookies"><strong>Cookies</strong></h3> <p>Cookies allow you to store information between browser sessions, and you can set their expiry date</p> <p>They contain:</p> <p>• A <em>name-value pair</em> containing the actual data.</p> <p>Cookie 保存在名称值对中 username = Bill Gates</p> <p>• An expiry date after which it is no longer valid.</p> <p>• The <em>domain and path of the server</em> it should be sent to</p> <p>JavaScript 可以用 <code>document.cookie</code> 属性创建、读取、删除 cookie。</p> <p>通过 JavaScript,可以这样创建 cookie:</p> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript">document<span class="token punctuation">.</span>cookie <span class="token operator">=</span> “version<span class="token operator">=</span>” <span class="token operator">+</span> <span class="token number">4</span><span class="token punctuation">;</span> document<span class="token punctuation">.</span>cookie <span class="token operator">=</span> “version<span class="token operator">=</span>” <span class="token operator">+</span> <span class="token function">encodeURIComponent</span><span class="token punctuation">(</span>document<span class="token punctuation">.</span>lastModified<span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token comment">//最后修改cookie的时机</span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span></span></code></pre> <ul> <li> <p>A cookie name cannot include semicolons,commas, or whitespace</p> <p>Hence the use of <strong>encodeURIComponent(value)</strong>.对 URI 组件进行编码。</p> <p>Must remember to <strong>decodeURIComponent()</strong> when reading the saved cookie 对 URI 组件进行解码。</p> <p>下次用户登陆时再次调用document.cookie获取信息</p> </li> </ul> <pre class="line-numbers language-javascript" data-language="javascript"><code class="language-javascript">document<span class="token punctuation">.</span>cookie <span class="token operator">=</span> “version<span class="token operator">=</span>” <span class="token operator">+</span> <span class="token function">encodeURIComponent</span><span class="token punctuation">(</span>document<span class="token punctuation">.</span>lastModified<span class="token punctuation">)</span> <span class="token operator">+</span> “ <span class="token punctuation">;</span> max<span class="token operator">-</span>age<span class="token operator">=</span>” <span class="token operator">+</span> <span class="token punctuation">(</span><span class="token number">60</span><span class="token operator">*</span><span class="token number">60</span><span class="token operator">*</span><span class="token number">24</span><span class="token operator">*</span><span class="token number">365</span><span class="token punctuation">)</span><span class="token punctuation">;</span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span></span></code></pre> <p>cookie 的名字(cname),cookie 的值(cvalue),以及知道 cookie 过期的天数(exdays)</p> <p>Note that <em>only</em> the name-value pair is stored in the name-value list associated with <strong>document.cookie</strong>.</p> <p>Attributes are stored by the system.</p> <pre class="line-numbers language-HTML" data-language="HTML"><code class="language-HTML"><html> <head><title>How many times have you been here before?</title> <script type="text/ javascript"> expireDate = new Date(); expireDate.setMonth(expireDate.getMonth()+6); hitCt = parseInt(cookieVal("pageHit")); hitCt++; document.cookie= "pageHit=" + hitCt + ";expires=" + expireDate.toGMTString(); function cookieVal(cookieName) { thisCookie = document.cookie.split("; "); //用;分割并返回数组 for (i=0; i<thisCookie.length; i++) { if (cookieName == thisCookie[i].split("=")[0])//找到名字为cookieName对应的值 return thisCookie[i].split("=")[1]; } return 0; } </script> </head> <body bgcolor="#FFFFFF"><h2> <script language="Javascript" type="text/javascript"> document.write("You have visited this page " + hitCt + " times."); </script></h2> </body> </html><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span></span></code></pre> <h3 id="A-Cautionary-Tale-SQL-Statements">**A Cautionary Tale: SQL Statements **</h3> <p><img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture/image-20221108225412740.png" alt="image-20221108225412740"></p> <p>前端输入 <strong>‘||’a’=‘a</strong>看似无意义,却正好拼成一个绝对成立的SQL语句</p> <p><img src="https://chilh-1311344212.cos.ap-beijing.myqcloud.com/picture/image-20221108225510101.png" alt="image-20221108225510101"></p> <p>用户非法获得后端数据</p> <h3 id="code">code</h3> <pre class="line-numbers language-html" data-language="html"><code class="language-html"><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>HTML</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>HEAD</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>TITLE</span><span class="token punctuation">></span></span>Forms<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>TITLE</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>SCRIPT</span><span class="token punctuation">></span></span><span class="token script"><span class="token language-javascript"> <span class="token keyword">var</span> helpArray <span class="token operator">=</span> <span class="token punctuation">[</span><span class="token string">"Enter your name in this input box."</span><span class="token punctuation">,</span> <span class="token string">"Enter your email address in this input box, \ in the format user@domain."</span><span class="token punctuation">,</span> <span class="token string">"Check this box if you liked our site."</span><span class="token punctuation">,</span> <span class="token string">"In this box, enter any comments you would \ like us to read."</span><span class="token punctuation">,</span> <span class="token string">"This button submits the form to the \ server-side script"</span><span class="token punctuation">,</span> <span class="token string">"This button clears the form"</span><span class="token punctuation">,</span> <span class="token string">"This TEXTAREA provides context-sensitive help. \ Click on any input field or use the TAB key to \ get more information about the input field."</span><span class="token punctuation">]</span><span class="token punctuation">;</span> <span class="token keyword">function</span> <span class="token function">helpText</span><span class="token punctuation">(</span><span class="token parameter">messageNum</span><span class="token punctuation">)</span> <span class="token punctuation">{</span> document<span class="token punctuation">.</span>myForm<span class="token punctuation">.</span>helpBox<span class="token punctuation">.</span>value <span class="token operator">=</span> helpArray<span class="token punctuation">[</span>messageNum<span class="token punctuation">]</span><span class="token punctuation">;</span> <span class="token comment">//myform是下面表单的名字,helpbox是最后定义的文本域</span> <span class="token punctuation">}</span> <span class="token keyword">function</span> <span class="token function">formSubmit</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span> window<span class="token punctuation">.</span>event<span class="token punctuation">.</span>returnValue <span class="token operator">=</span> <span class="token boolean">false</span><span class="token punctuation">;</span> <span class="token comment">//默认不提交</span> <span class="token keyword">if</span> <span class="token punctuation">(</span><span class="token function">confirm</span><span class="token punctuation">(</span><span class="token string">"Are you sure you want to submit?"</span><span class="token punctuation">)</span><span class="token punctuation">)</span> <span class="token comment">//windows.confirm()弹出带有确认和取消的对话框,返回true/false</span> window<span class="token punctuation">.</span>event<span class="token punctuation">.</span>returnValue <span class="token operator">=</span> <span class="token boolean">true</span><span class="token punctuation">;</span> <span class="token comment">// so in this case it now performs the action</span> <span class="token punctuation">}</span> <span class="token keyword">function</span> <span class="token function">formReset</span><span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token punctuation">{</span> window<span class="token punctuation">.</span>event<span class="token punctuation">.</span>returnValue <span class="token operator">=</span> <span class="token boolean">false</span><span class="token punctuation">;</span> <span class="token keyword">if</span> <span class="token punctuation">(</span><span class="token function">confirm</span><span class="token punctuation">(</span><span class="token string">"Are you sure you want to reset?"</span><span class="token punctuation">)</span><span class="token punctuation">)</span> window<span class="token punctuation">.</span>event<span class="token punctuation">.</span>returnValue <span class="token operator">=</span> <span class="token boolean">true</span><span class="token punctuation">;</span> <span class="token punctuation">}</span> </span></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>SCRIPT</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>HEAD</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>BODY</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>FORM</span> <span class="token attr-name">NAME</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>myForm<span class="token punctuation">"</span></span> <span class="token special-attr"><span class="token attr-name">ONSUBMIT</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">formSubmit</span><span class="token punctuation">(</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span> <span class="token attr-name">ACTION</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span>http://localhost/cgi-bin/mycgi</span> <span class="token special-attr"><span class="token attr-name">ONRESET</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token keyword">return</span> <span class="token function">formReset</span><span class="token punctuation">(</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span><span class="token punctuation">></span></span> Name: <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>INPUT</span> <span class="token attr-name">TYPE</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>text<span class="token punctuation">"</span></span> <span class="token attr-name">NAME</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>name<span class="token punctuation">"</span></span> <span class="token special-attr"><span class="token attr-name">ONFOCUS</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">helpText</span><span class="token punctuation">(</span><span class="token number">0</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span> <span class="token special-attr"><span class="token attr-name">ONBLUR</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">helpText</span><span class="token punctuation">(</span><span class="token number">6</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>BR</span><span class="token punctuation">></span></span> Email: <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>INPUT</span> <span class="token attr-name">TYPE</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>text<span class="token punctuation">"</span></span> <span class="token attr-name">NAME</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>email<span class="token punctuation">"</span></span> <span class="token special-attr"><span class="token attr-name">ONFOCUS</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">helpText</span><span class="token punctuation">(</span><span class="token number">1</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span> <span class="token special-attr"><span class="token attr-name">ONBLUR</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">helpText</span><span class="token punctuation">(</span><span class="token number">6</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>BR</span><span class="token punctuation">></span></span> Click here if you like this site <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>INPUT</span> <span class="token attr-name">TYPE</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>checkbox<span class="token punctuation">"</span></span> <span class="token attr-name">NAME</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>like<span class="token punctuation">"</span></span> <span class="token special-attr"><span class="token attr-name">ONFOCUS</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">helpText</span><span class="token punctuation">(</span><span class="token number">2</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span> <span class="token special-attr"><span class="token attr-name">ONBLUR</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">helpText</span><span class="token punctuation">(</span><span class="token number">6</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>BR</span><span class="token punctuation">></span></span> Any comments?<span class="token tag"><span class="token tag"><span class="token punctuation"><</span>BR</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>TEXTAREA</span> <span class="token attr-name">NAME</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>comments<span class="token punctuation">"</span></span> <span class="token attr-name">ROWS</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> 5</span> <span class="token attr-name">COLS</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> 45</span> <span class="token special-attr"><span class="token attr-name">ONFOCUS</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">helpText</span><span class="token punctuation">(</span><span class="token number">3</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span> <span class="token special-attr"><span class="token attr-name">ONBLUR</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">helpText</span><span class="token punctuation">(</span><span class="token number">6</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>TEXTAREA</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"><</span>BR</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>INPUT</span> <span class="token attr-name">TYPE</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>submit<span class="token punctuation">"</span></span> <span class="token attr-name">VALUE</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>Submit<span class="token punctuation">"</span></span> <span class="token special-attr"><span class="token attr-name">ONFOCUS</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">helpText</span><span class="token punctuation">(</span><span class="token number">4</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span> <span class="token special-attr"><span class="token attr-name">ONBLUR</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">helpText</span><span class="token punctuation">(</span><span class="token number">6</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>INPUT</span> <span class="token attr-name">TYPE</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>reset<span class="token punctuation">"</span></span> <span class="token attr-name">VALUE</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>Reset<span class="token punctuation">"</span></span> <span class="token special-attr"><span class="token attr-name">ONFOCUS</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">helpText</span><span class="token punctuation">(</span><span class="token number">5</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span> <span class="token special-attr"><span class="token attr-name">ONBLUR</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value javascript language-javascript"><span class="token function">helpText</span><span class="token punctuation">(</span><span class="token number">6</span><span class="token punctuation">)</span></span><span class="token punctuation">"</span></span></span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>TEXTAREA</span> <span class="token attr-name">NAME</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span>helpBox<span class="token punctuation">"</span></span> <span class="token special-attr"><span class="token attr-name">STYLE</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> <span class="token punctuation">"</span><span class="token value css language-css"><span class="token property">position</span><span class="token punctuation">:</span> absolute<span class="token punctuation">;</span> <span class="token property">right</span><span class="token punctuation">:</span>0<span class="token punctuation">;</span> <span class="token property">top</span><span class="token punctuation">:</span> 0</span><span class="token punctuation">"</span></span></span> <span class="token attr-name">ROWS</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> 4</span> <span class="token attr-name">COLS</span> <span class="token attr-value"><span class="token punctuation attr-equals">=</span> 45</span><span class="token punctuation">></span></span> This TEXTAREA provides context-sensitive help. Click on any input field or use the TAB key to get more information about the input field.<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>TEXTAREA</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"></</span>FORM</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>BODY</span><span class="token punctuation">></span></span><span class="token tag"><span class="token tag"><span class="token punctuation"></</span>HTML</span><span class="token punctuation">></span></span><span aria-hidden="true" class="line-numbers-rows"><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span><span></span></span></code></pre>