3.3-javaBean
JSPs and JavaBeans
JSPs allow static HTML to be mixed with dynamically generated content from servlets.
– Helps to create presentation on the server side more easily.
– Helps to separate presentation from content.
JSP全称Java Server Pages,是一种动态网页开发技术。它使用JSP标签在HTML网页中插入Java代码。标签通常以<%开头以%>结束。
JSP是一种Java servlet,主要用于实现Java web应用程序的用户界面部分。网页开发者们通过结合HTML代码、XHTML代码、XML元素以及嵌入JSP操作和命令来编写JSP。
JSP的优势
以下列出了使用JSP带来的其他好处:
- 与ASP相比:JSP有两大优势。首先,动态部分用Java编写,而不是VB或其他MS专用语言,所以更加强大与易用。第二点就是JSP易于移植到非MS平台上。
- 与纯 Servlets相比:JSP可以很方便的编写或者修改HTML网页而不用去面对大量的println语句。
- 与SSI相比:SSI无法使用表单数据、无法进行数据库链接。
- 与JavaScript相比:虽然JavaScript可以在客户端动态生成HTML,但是很难与服务器交互,因此不能提供复杂的服务,比如访问数据库和图像处理等等。
- 与静态HTML相比:静态HTML不包含动态信息。
JSP基础
On a web server, JSP files are often stored in the same directory as HTML files
脚本程序可以包含任意量的Java语句、变量、方法或表达式,只要它们在脚本语言中是有效的。
脚本程序的语法格式:
<% 代码片段 %>
任何文本、HTML标签、JSP元素必须写在脚本程序的外面。
第二部中的转换:这种转化只是简单地将所有模板文本改用println()语句,并且将所有的JSP元素转化成Java代码。
How the servlet is created from the JSP
The HTML and JSP code forms the void _jspService() method of the new servlet, apart from JSP declarations
JSP 生命周期
-
编译阶段:
servlet容器编译servlet源文件,生成servlet类。如果这个文件没有被编译过,或者在上次编译后被更改过,则编译这个JSP文件。
-
初始化阶段:
加载与JSP对应的servlet类,创建其实例,并调用它的初始化方法。
如果您需要执行自定义的JSP初始化任务,复写jspInit()方法就行了,就像下面这样:
public void jspInit(){ // 初始化代码 }
程序只初始化一次
-
执行阶段:
调用与JSP对应的servlet实例的服务方法
当JSP网页完成初始化后,JSP引擎将会调用_jspService()方法。
_jspService()方法需要一个HttpServletRequest对象和一个HttpServletResponse对象作为它的参数,就像下面这样:
void _jspService(HttpServletRequest request,HttpServletResponse response) { // 服务端处理代码 }
-
销毁阶段:
调用与JSP对应的servlet实例的销毁方法,然后销毁servlet实例
当您需要执行任何清理工作时复写jspDestroy()方法,比如释放数据库连接或者关闭文件夹等等。
很明显,JSP生命周期的四个主要阶段和servlet生命周期非常相似,下面给出图示:
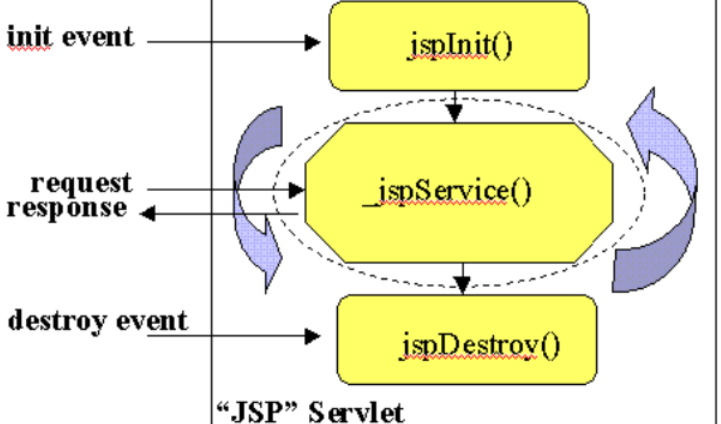
syntax
scripting elements which let you specify code that will be part of the resultant servlet;
directives which let you control the overall structure of the servlet;
用来设置与整个JSP页面相关的属性。
actions它能够动态插入一个文件,重用JavaBean组件,引导用户去另一个页面,为Java插件产生相关的HTML等等。
在servlet中相当于html 语法被out.print()包住,而JSP中HTML直接写,相当于Java语句被不同类型的JSP包住。
Scripting elements
insert code into the resulting servlet in three ways:
– expressions <%= expression %>
– scriptlets <% code %> 脚本程序可以包含任意量的Java语句、变量、方法或表达式
– declarations <%! code %> 声明一个或多个变量、方法
• Comments:
<%-- JSP Comment (won’t appear in source)–%>
<!-– HTML Comment (will appear in source)–>
Each element has also an alternative XML syntax.
JSP expressions
Current time: <%= java.util.Date() %>
• The expression is evaluated, converted into a string, and inserted in the page (where it appears).
将表达式的计算结果转换为字符串并插入回原来的位置
在运行时执行计算:-拥有对请求信息的完全访问权限
表达式内部不能定义变量
To simplify expressions, you can use a number of predefined variables (aka implicit objects) e.g. request, response, session, out
JSP支持九个自动定义的变量,江湖人称隐含对象。这九个隐含对象的简介见下表:
对象 | 描述 |
---|---|
request | HttpServletRequest类的实例 |
response | HttpServletResponse类的实例 |
out | PrintWriter类的实例,用于把结果输出至网页上 |
session | HttpSession类的实例 automatically created |
application | ServletContext类的实例,与应用上下文有关 |
config | ServletConfig类的实例 |
pageContext | PageContext类的实例,提供对JSP页面所有对象以及命名空间的访问 |
page | 类似于Java类中的this关键字 |
Exception | Exception类的对象,代表发生错误的JSP页面中对应的异常对象 |
-
application: This is the ServletContext object that allows you to share data with other servlets and JSPs:
Attributes set in application are accessible to all servlets/JSPs in a web application.
Useful when some of the servlets are not part of a session or don’t need to maintain session
web应用程序中需要由所有servlet共享的信息片段(通常很小)可以在ServletContext对象中设置。
-
pageContext**: This is the **PageContext object which gives a single point of access to many of the page attributes
如果一个方法或构造函数需要多个与页面相关的对象(request, response, out,…)传递pageContext更容易
也可以编写与之等价的XML语句:
<jsp:expression>
表达式
</jsp:expression>
JSP 表达式不能以分号结尾
<jsp:setProperty name=“user”
property=“id”
value=‘<%= “UserID” + Math.random() %>’ />
JSP scriptlets
Scriplets are used to insert arbitrary Java code:
– they have access to the same pre-defined variables
其中写的内容会翻译在Servlet的Service方法中,可以在Service方法中定义局部变量或者调用其他方法,但是不能在Service中再定义其他的方法,也就是可以在<% %>中定义局部变量或者调用方法,但不能定义方法。
Scriptlet code gets inserted directly into a servlet’s _jspServicemethod, so they can be used for:
– setting response headers and status codes;
<% response.setContentType(“application/vnd.ms-excel”); %>
– invoking side effects, e.g. writing to server log or updating a database;
– executing code containing loops, conditionals or other “complex” constructs.
<%
String queryData = request.getQueryString();
out.println(“Attached Get data is: ” + queryData);
%>
scriplets are much more powerful than expressions
可以编写与其等价的XML语句,就像下面这样:
<jsp:scriptlet>
代码片段
</jsp:scriptlet>
HTML中的写法:
• Static HTML is inserted as out.println() statements.
会将HTML自动转为 println中内容
• So we can write JSP code that looks like this:
<% if (Math.random()<0.5) { %>
Have a <b>nice</b> day!
<% } else { %>
Have a <b>lousy<b> day!
<% } %>
转化到servlet中长这样
if (Math.random()<0.5) {
out.println(“Have a <b>nice</b> day!”);
}
else { out.println(“Have a <b>lousy<b> day!”); }
JSP declarations
声明语句在class中而不在 **_**jspService method中
<%! private int accessCount = 0; %> declaration +initialisation
Accesses to page since last server reboot:
<% ++accessCount; %> scriptlet
<%= accessCount %> expression
Since declarations appear in the class body of resultant servlet, they become instance variables shared by multiple requests. May need to synchronize access!
编写与其等价的XML语句,就像下面这样:
<jsp:declaration>
代码片段
</jsp:declaration>
JSP 声明中定义的变量、方法和类(内部类)是全局性的,在 JSP 页面中的任何地方都能够使用。JSP 声明中不能使用out.print()系列方法输出操作。

例子:写出返回页面
JSP directives
JSP指令用来设置与整个JSP页面相关的属性
<%@ directive attribute = ‘value’ %>
<%@ directive attribute1 = “value1”
attribute2 = “value2”
// ...
attributeN = “valueN” %>
必须用引号! 如果想单用引号**\”**
这里有三种指令标签:
指令 | 描述 |
---|---|
<%@ page … %> | 定义页面的依赖属性,比如脚本语言、error页面、缓存需求等等 |
<%@ include … %> | 包含其他文件 |
<%@ taglib … %> | 引入标签库的定义,可以是自定义标签 |
page directive: lets you control structure by importing classes, customising a superclass, setting content type, etc. Can be placed anywhere inside the JSP document.
– include directive: lets you insert a file into the JSP at translation time. It’s placed where you want the file to be inserted.
Attributes of the page directive
import: lets you import packages.
<%@ page import = “package.class” %>
<%@ page import = “package.class1, ..., package.classN” %>
<%@ page import = “java.util.*” %>
contentType: sets content type in the response header.
<%@ page contentType = “text/plain” %>
JSPs for regular “text/html” servlets is default MIME type for
session: controls whether page participates in HTTP sessions
<%@ page session = “true” %> <%-- Default --%>
<%@ page session = “false” %>
当为true时,预定义变量session被绑定到现有会话,如果不存在,则创建一个新会话
buffer: specifies size of buffer used by the out variable.
<%@ page buffer = “sizekb” %>
<%@ page buffer = “none” %>
autoflush: controls whether buffer is automatically emptied when full
<%@ page autoflush = “true” %> <%-- Default --%>
<%@ page autoflush = “false” %>
errorPage如果希望在当前页面运行时出现错误时,指定一个错误提示页面,那么errorPage
属性告诉JSP引擎显示哪个页面。errorPage
属性的值是相对URL。
当抛出所有未捕获的异常时,以下指令用于在页面出错时指定显示MyErrorPage.jsp
的内容 -
<%@ page errorPage = “Relative URL” %>
isErrorPage
indicates whether or not the current page can act as an error page for another JSP page.
isErrorPage
的值可为true
或false
。 isErrorPage
属性的默认值为false
。
例如,handleError.jsp
将isErrorPage
选项设置为true
,因为它应该处理错误
<%@ page isErrorPage = “true” %>
<%@ page isErrorPage = “false” %> <%-- Default -->
extends: indicates the superclass of the servlet (not often used)
info: defines a string retrievable through getServletInfo.
language: defines language used in the page.
– “java” is both the default and only legal choice!
xml版本
XML versions
<jsp:directive.directiveType attribute = “value” />
<%@ page import = “java.util.Date” %>
<jsp:directive.page import = “java.util.Date” />
JSP actions
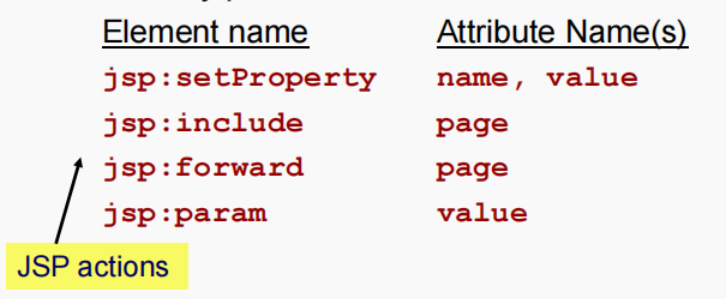
<jsp:setProperty name=“user”
property=“id”
value=‘<%= “UserID” + Math.random() %>’ />