DSD-2.5-FIFO and LIFO Buffers
FIFO and LIFO Buffers
First-In First-Out Buffer (FIFO)
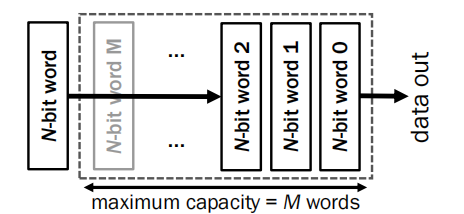
• It is digital device/block to regulate flow of data received in irregular time intervals
• Data can be read while the data fill up the buffer
M x N-bit (or simply M x N) FIFO indicates a capacity of M data word, N-bit each.
可以存M个Nbit的字符
External Interface
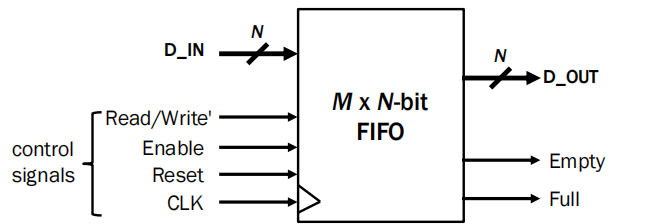
entity FIFO_MN is
generic (N: positive := 4; M: positive := 4);
port ( D_IN: in std_logic_vector(N-1 downto 0);
Read_WriteN: in std_logic;
Enable: in std_logic;
Reset: in std_logic;
CLK: in std_logic;
D_OUT: out std_logic_vector(N-1 downto 0);
Empty, Full: out std_logic);
end FIFO_MN;
GENERIC(常数名 数据类型 := 设定值);
-
定义实体的端口大小;
-
设计实体的物理特征;
在例化时应该加generic map来修改不同需求下的元件例化参数
利用移位寄存器来设计FIFO
Suppose we want to implement a M x N FIFO, it is clearly that we will need at least M x N D flip flops to store the bits.
we make use of the N M-bit shift register to build the FIFO
我们使用N个M比特的移位寄存器来构建FIFIO
-
when a new word is added, each of the bits in this word will be shifted into the N shift registers separately.
-
the older words are also shifted, therefore it is important that we remember where the oldest word is by a counter of a suitable size.
If it is full, we should read the output (and free the space) before we can receive more word(s); otherwise the buffer overflows
Overflow a buffer should be avoided at all costs. i.e. don’t write when Full = 1
Dout一直在读,R/W’直影响计数器加减以及移位寄存器保持还是移动
之所以把计数器初始化为1111,是为了统一计数器和shifer的索引,这样可以保证索引始终指在最后一个有效数字上。且由于M=4所以
Last-In First-Out Buffer (LIFO)
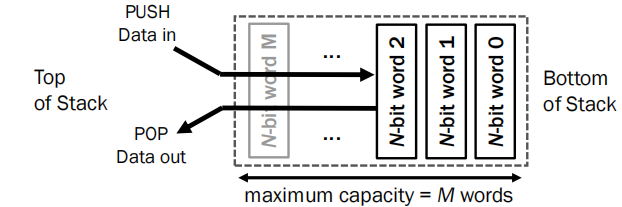
push-down stack.
• Data words are pushed down into the buffer.
• The last word that goes into the stack (push) will be the first one be taken out (pop).
PUSH place a word onto the top of stack
POP remove a word from the top of stack
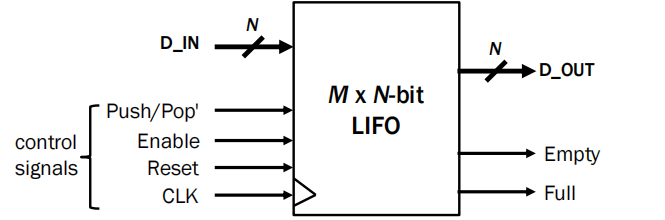
entity LIFO_MN is
generic (N: positive := 4; M: positive := 4);
port ( D_IN: in std_logic_vector(N-1 downto 0);
Push_PopN, Enable, Reset: in std_logic;
CLK: in std_logic;
D_OUT: out std_logic_vector(N-1 downto 0);
Empty, Full: out std_logic);
end LIFO_MN;
shift register has to shift to both left and right, so that after a word is shifted in to the right (push), it can be shifted out to the left (pop, last in first out).
use N M-bit bi-directional shift registers to build the LIFO
-
when a new word is added (push), each of the bits will be shifted right into the N shift registers in parallel. At the same time, the older words are all shifted right.
-
the top of the stack is always at the leftmost.
因为这里读数据只读第一个位置,索引与计数器无关(不随计数器变化),所以计数器不用初始化为1111;FIFO和LIFO区别就在于索引动不动,其他没有本质区别
When it is full, we should pop data out before we push more words in ; otherwise the buffer overflows, which means that bottom of the stack - AAword0 is lost permanently